import gym env = gym.make("MountainCar-v0") env.reset() done = Falsewhile not done: action = 2 # always go right! env.step(action) env.render()
8 Haziran 2020 Pazartesi
Q LEARNING
5 Haziran 2020 Cuma
Autonomous Car Simulation with CARLA
D:\Carla\PythonAPI\examples>python3.7 Selfdriving_Study1.py
ActorBlueprint(id=vehicle.tesla.model3,tags=[vehicle, tesla, model3])
destroying actors
done.
import glob import os import sys try: sys.path.append(glob.glob('../carla/dist/carla-*%d.%d-%s.egg' % ( sys.version_info.major, sys.version_info.minor, 'win-amd64' if os.name == 'nt' else 'linux-x86_64'))[0]) except IndexError: passimport carla import random import time import numpy as np import cv2 IM_WIDTH = 640IM_HEIGHT = 480 def process_img(image): i = np.array(image.raw_data) i2 = i.reshape((IM_HEIGHT, IM_WIDTH, 4)) i3 = i2[:, :, :3] cv2.imshow("", i3) cv2.waitKey(1) return i3/255.0 actor_list = [] try: client = carla.Client('localhost', 2000) client.set_timeout(10.0) world = client.get_world() blueprint_library = world.get_blueprint_library() bp = blueprint_library.filter('model3')[0] print(bp) spawn_point = random.choice(world.get_map().get_spawn_points()) vehicle = world.spawn_actor(bp, spawn_point) vehicle.apply_control(carla.VehicleControl(throttle=1.0, steer=0.0)) # vehicle.set_autopilot(True) # if you just wanted some NPCs to drive. actor_list.append(vehicle) # https://carla.readthedocs.io/en/latest/cameras_and_sensors # get the blueprint for this sensor blueprint = blueprint_library.find('sensor.camera.rgb') # change the dimensions of the image blueprint.set_attribute('image_size_x', f'{IM_WIDTH}') blueprint.set_attribute('image_size_y', f'{IM_HEIGHT}') blueprint.set_attribute('fov', '110') # Adjust sensor relative to vehicle spawn_point = carla.Transform(carla.Location(x=2.5, z=0.7)) # spawn the sensor and attach to vehicle. sensor = world.spawn_actor(blueprint, spawn_point, attach_to=vehicle) # add sensor to list of actors actor_list.append(sensor) # do something with this sensor sensor.listen(lambda data: process_img(data)) time.sleep(5) finally: print('destroying actors') for actor in actor_list: actor.destroy() print('done.')
import glob import os import sys try: sys.path.append(glob.glob('../carla/dist/carla-*%d.%d-%s.egg' % ( sys.version_info.major, sys.version_info.minor, 'win-amd64' if os.name == 'nt' else 'linux-x86_64'))[0]) except IndexError: passimport carla import random import time import numpy as np import cv2 im_width = 640im_height = 480 def process_img(image): i = np.array(image.raw_data) i2 = i.reshape((im_height, im_width, 4)) i3 = i2[:, :, :3] cv2.imshow("", i3) cv2.waitKey(1) return i3/255.0 actor_list = [] try: client = carla.Client('localhost', 2000) client.set_timeout(10.0) world = client.get_world() blueprint_library = world.get_blueprint_library() bp = blueprint_library.filter('model3')[0] print(bp) spawn_point = random.choice(world.get_map().get_spawn_points()) vehicle = world.spawn_actor(bp, spawn_point) vehicle.apply_control(carla.VehicleControl(throttle=1.0, steer=0.0)) actor_list.append(vehicle) # sleep for 5 seconds, then finish: time.sleep(5) finally: print('destroying actors') for actor in actor_list: actor.destroy() print('done.')
Kaydol:
Kayıtlar (Atom)
Ros2 çalışmaları
1) Her saniye yazı yazdırma. Eklediğim kod öncelikle Hello Cpp Node yazdıracak ardınca Hello ekleyecek. benim .cpp dosyamın adı my_first_no...
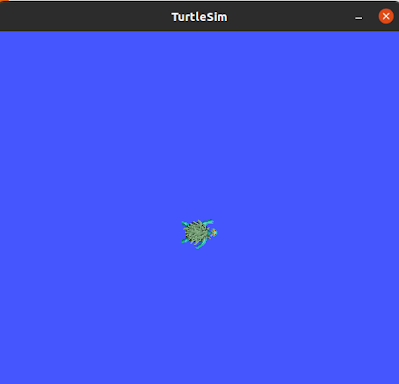
-
Udemy Problems Solve Exercise-1 Quiz: Average Electricity Bill It's time to try a calculation in Python! My electricity bil...
-
I've started programming python but Education videos didn't help me for learning and remembering python command. That's w...